The Life Cycle of Applet in Java with Example
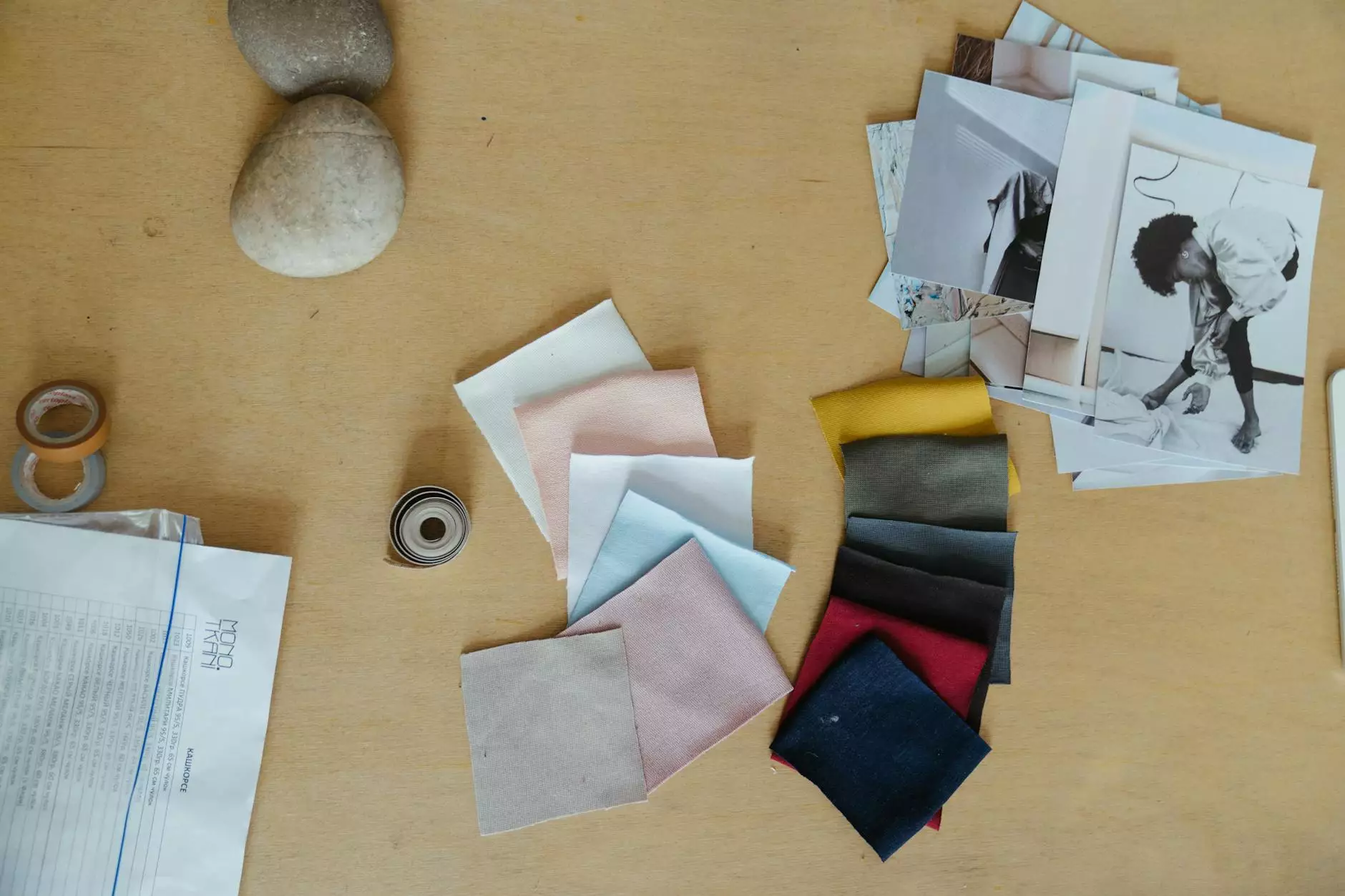
Introduction
In the world of modern technology, Java applets play a significant role in enhancing user experiences through interactive web applications. Understanding the life cycle of applets in Java is crucial for developers to create robust and efficient applications. In this comprehensive guide, we will explore the different stages of the applet life cycle and provide examples to illustrate their functionality.
What is an Applet?
An applet is a small program, written in Java programming language, that runs within a web browser. It can be embedded within an HTML page and executed when the page is loaded. Applets offer dynamic interaction, such as animations, games, and data processing, enhancing the user experience on webpages.
The Life Cycle of an Applet
Understanding the life cycle of an applet is crucial for developers to implement the appropriate methods and ensure proper functionality. The following sections outline the different stages of the applet life cycle:
1. Initialization: init() Method
When an applet begins its execution, it goes through the initialization stage. In this stage, the Java Virtual Machine (JVM) creates an instance of the applet and calls the init() method. The init() method is responsible for initializing the applet's variables, loading resources, and setting initial states. It is executed only once throughout the applet's lifetime.
2. Start: start() Method
After the initialization stage, the applet moves to the start stage. Here, the start() method is called by the JVM. The start() method is used to start the execution of the applet's code. It is called every time the applet needs to be visually displayed or when the applet resumes execution after being stopped.
3. Execution: paint() Method
Once the applet has been initialized and started, the execution stage begins. In this stage, the paint() method is called by the JVM. The paint() method is responsible for rendering the applet's visual representation on the screen. It is called automatically whenever the applet needs to be redrawn, such as when it first appears on the screen or when it is resized.
4. Stop: stop() Method
If an applet needs to be stopped, perhaps due to the user navigating away from the webpage or closing the browser, the stop stage is entered. The stop() method is called by the JVM to pause the execution of the applet's code. Cleaning up resources or stopping any ongoing operations typically occurs in this stage.
5. Destroy: destroy() Method
Finally, when the applet is no longer needed, the destroy stage is reached. The destroy() method is called by the JVM to release any resources held by the applet and perform final cleanup operations. This method is executed just before the applet's instance is permanently removed from memory.
Example: Calculating the Fibonacci Sequence
To demonstrate the applet life cycle, let's consider an example where we create an applet to calculate the Fibonacci sequence.
Implementation
Here is a basic implementation of an applet that calculates and displays the Fibonacci sequence:
import java.applet.*; import java.awt.*; public class FibonacciApplet extends Applet { int prev = 0; int next = 1; int result; public void init() { // Initialization code } public void start() { // Start code } public void paint(Graphics g) { // Paint code } public void stop() { // Stop code } public void destroy() { // Destruction code } }Explanation
In the above example, we create a class called FibonacciApplet that extends the java.applet.Applet class. Within this class, we define variables to store the previous and next Fibonacci numbers, as well as the result of the calculation.
The init(), start(), paint(), stop(), and destroy() methods are also implemented, providing space for additional functionality during each stage of the applet's life cycle.
Testing the Applet
To test the applet, we can embed it within an HTML page. Here is an example:
Fibonacci AppletWhen loading the HTML page, the applet's life cycle will be triggered, and the Fibonacci sequence will be calculated and displayed on the screen.
Conclusion
In this comprehensive guide, we have explored the life cycle of an applet in Java and provided an example of a Fibonacci sequence calculator applet. Understanding the various stages within an applet's life cycle is essential for creating efficient and interactive web applications.
By following the best practices and leveraging the power of Java applets, businesses in the Doctors, Health & Medical, and Dentists categories can enhance their online presence and offer seamless user experiences.
For more information and assistance, feel free to reach out to us at ebhor.com. We are here to help you optimize your web development strategies and create remarkable applications.
life cycle of applet in java with example